sindresorhus/conf

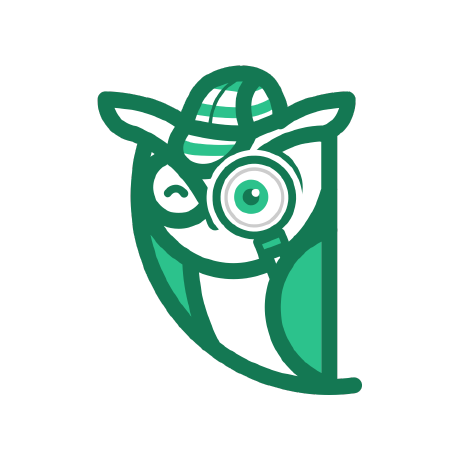








Do you want to work on this issue?
You can request for a bounty in order to promote it!
Defaults not applied from schema #85
YodaDaCoda posted onGitHub
I'm using a schema to create my settings, and I've been unable to have conf
or electron-config
use the defaults provided in the schema.
I've included a reduced test case here:
const Conf = require('conf');
const schema = {
fizz: {
type: 'string',
default: 'buzz',
},
foo: {
type: 'object',
properties: {
bar: {
type: 'string',
default: 'baz',
},
},
},
};
const config = new Conf({schema});
console.log(config.get('fizz')); // undefined - should be 'buzz'
console.log(config.get('foo')); // undefined - should be { bar: 'baz' }
console.log(config.get('foo.bar')); // undefined - should be 'baz'
console.assert(config.get('fizz') === 'buzz'); // Assertion failed
console.assert(config.get('foo.bar') === 'baz'); // Assertion failed
An rough solution to populate defaults from schema is below, but I wouldn't expect to have to do this when using a schema that includes defaults.
function setDefaults(schema, config, key_chain) {
for (let [key, val] of Object.entries(schema)) {
let this_key = (key_chain ? key_chain + '.' : '') + key;
if (val.type === 'object') {
return setDefaults(val.properties, config, this_key);
}
if (!config.has(key) && Object.hasOwnProperty.call(val, 'default')) {
config.set(this_key, val.default);
}
}
}