lc-soft/trad



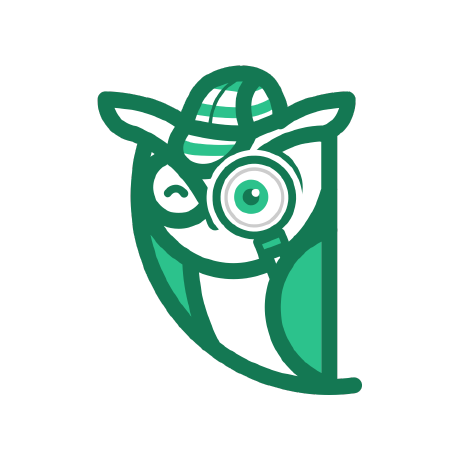

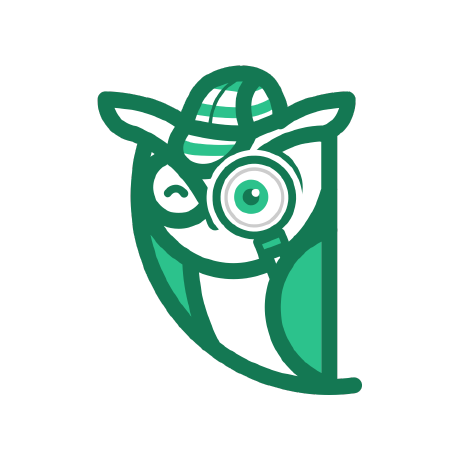
The issue has been solved
Class method naming style #1
lc-soft posted onGitHub
Description:
Class instance methods and static methods have the same naming style and are easy to conflict.
For example:
class MyDict {
fetchValue() {}
static createStringKeyDict() {}
static createIntKeyDict() {}
}
Output:
typedef struct MyDictRec_* MyDict;
void *MyDict_FetchValue(MyDict);
Dict MyDict_CreateStringKeyDict();
Dict MyDict_CreateIntKeyDict();
MyDict MyDict_New();
Reference:
Cost:
30 miutes
benefits:
Make class instance methods and static methods in C code easier to identify
Assignee:
Who do you want to be assignee for this feature?
- I will implement this feature
- Other contributors
- Maintainers
- I don't know
Contribution:
For this feature, I will provide these contributions:
- Contribute documentions
- Contribute testcases
- Develop other feuatres, or fix existing bugs
- Write an article to promote this project
- Fund this issue on IssueHunt
- Donate this project on OpenCollective
- I don't want to provide any help